In this post, I will show you the basics of Processing(www.processing.org), the free and open source Java/C++-based coding graphics environment. For those of you familiarized with Arduino, the robotics microcontroller, its coding environment is inspired by Processing, so it will be easy to use! This software allows you to use straighforward syntax from simple circle shapes to complex 3D OpenGL commands.
The thing I particularly like abut this is the easy installation of a library called OpenCV, a camera-based image processing library that allows face detection and hand tracking, combined with the easy syntax of Processing. OpenCV is good for robots as it could track colors precisely and easily interact with the human. I will describe this in a futute post.
First, I will get you familiarized with the coding enviroment.
To get started, download it from www.processing.org.
The app will appear in downloads, so drag it to the Applications folder.
After launching, this is what the software should look like:
Now try some code, and copy-paste this in the console:
ellipse(50,50,80,80); //Circle at x,50 y,50 l,80 w,80
//Everything in this font is code!!!
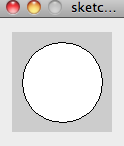
Syntax:
ellipse(x(pixels)coordinate, y(pixels)coordinate, length, width);
This draws a circle, 50 pixels x-coordinate(1st number), 50 pixels y-coordinate(2nd number), 80 pixels length(3rd number),
80 pixels width(4th number). The doubleslash "//" is a comment, and this allows you to put non-exactable words to remind you what this piece or line of code does.
Download the software at processing.org, copy/paste the following, and run it with the "play" symbol. After ~10 seconds, a window pops up, with a white circle that has poor image quality.
To smoothen the rugged circle, just add the command smooth().
Try this:
smooth(); //Smoothen circle
ellipse(50,50,80,80);
This draws a much smoother circle!
If you want to smooth only one object, and the other one non-smooth, use noSmooth() :
smooth();
ellipse(50,50,80,80);
noSmooth();
ellipse(40,40,20,20);
Filling a object is easy: fill();
Syntax:
fill(red value, green value, blue value);
or fill(brightness); (for black and white)
fill(255,255,0);//rgb mode
ellipse(50,50,80,80);
The fill() takes a value only from 0-255. 0 is the darkest for each color value/black (r,g,b) and 255 is white. You combine these primary colors to create secondary colors of the rainbow/visible color spectrum. Play with these values to create rainbow colors!
I won't cover everything about Processing in this post, because you can find tutorials at http://processing.org/tutorials/. My objective here is to make you familiarized for future codes I post for graphics and animations and cool sketches, and some OpenCV stuff in processing.